World class More...
#include <stage.hh>
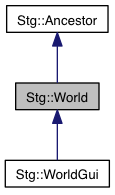
Classes | |
class | Event |
struct | ltx |
struct | lty |
Public Member Functions | |
virtual void | AddModel (Model *mod) |
void | AddModelName (Model *mod, const std::string &name) |
void | AddPowerPack (PowerPack *pp) |
SuperRegion * | AddSuperRegion (const point_int_t &coord) |
void | AddUpdateCallback (world_callback_t cb, void *user) |
void | CancelQuit () |
void | CancelQuitAll () |
void | ClearRays () |
virtual std::string | ClockString (void) const |
void | ConsumeQueue (unsigned int queue_num) |
Model * | CreateModel (Model *parent, const std::string &typestr) |
SuperRegion * | CreateSuperRegion (point_int_t origin) |
void | DestroySuperRegion (SuperRegion *sr) |
void | DisableEnergy (Model *m) |
void | EnableEnergy (Model *m) |
void | Enqueue (unsigned int queue_num, usec_t delay, Model *mod, model_callback_t cb, void *arg) |
void | Extend (point3_t pt) |
const std::set< Model * > | GetAllModels () const |
unsigned int | GetEventQueue (Model *mod) const |
const bounds3d_t & | GetExtent () const |
Model * | GetGround () |
Model * | GetModel (const std::string &name) const |
SuperRegion * | GetSuperRegion (const point_int_t &org) |
SuperRegion * | GetSuperRegionCreate (const point_int_t &org) |
uint64_t | GetUpdateCount () const |
Worldfile * | GetWorldFile () |
virtual bool | IsGUI () const |
virtual void | Load (const std::string &worldfile_path) |
void | LoadBlock (Worldfile *wf, int entity) |
void | LoadBlockGroup (Worldfile *wf, int entity) |
void | LoadModel (Worldfile *wf, int entity) |
void | LoadSensor (Worldfile *wf, int entity) |
void | Log (Model *mod) |
void | MapPoly (const std::vector< point_int_t > &poly, Block *block, unsigned int layer) |
int32_t | MetersToPixels (meters_t x) const |
point_int_t | MetersToPixels (const point_t &pt) const |
void | NeedRedraw () |
bool | PastQuitTime () |
bool | Paused () const |
virtual void | PopColor () |
virtual void | PushColor (Color col) |
virtual void | PushColor (double r, double g, double b, double a) |
void | Quit () |
void | QuitAll () |
RaytraceResult | Raytrace (const Ray &ray) |
RaytraceResult | Raytrace (const Pose &pose, const meters_t range, const ray_test_func_t func, const Model *finder, const void *arg, const bool ztest) |
void | Raytrace (const Pose &pose, const meters_t range, const radians_t fov, const ray_test_func_t func, const Model *finder, const void *arg, RaytraceResult *samples, const uint32_t sample_count, const bool ztest) |
virtual Model * | RecentlySelectedModel () const |
void | RecordRay (double x1, double y1, double x2, double y2) |
virtual void | Redraw (void) |
void | RegisterOption (Option *opt) |
Register an Option for pickup by the GUI. | |
virtual void | Reload () |
virtual void | RemoveModel (Model *mod) |
void | RemovePowerPack (PowerPack *pp) |
int | RemoveUpdateCallback (world_callback_t cb, void *user) |
double | Resolution () const |
virtual bool | Save (const char *filename) |
void | ShowClock (bool enable) |
Control printing time to stdout. | |
usec_t | SimTimeNow (void) const |
virtual void | Start () |
virtual void | Stop () |
bool | TestQuit () const |
virtual void | TogglePause () |
void | TryCharge (PowerPack *pp, const Pose &pose) |
virtual void | UnLoad () |
virtual bool | Update (void) |
uint64_t | UpdateCount () |
World (const std::string &name="MyWorld", double ppm=DEFAULT_PPM) | |
virtual | ~World () |
Static Public Member Functions | |
static void | Run () |
static void * | update_thread_entry (std::pair< World *, int > *info) |
static bool | UpdateAll () |
Public Attributes | |
std::set< Model * > | active_energy |
std::set< ModelPosition * > | active_velocity |
std::vector < std::priority_queue< Event > > | event_queues |
Model * | ground |
bool | paused |
if true, the simulation is stopped | |
std::vector< std::queue< Model * > > | pending_update_callbacks |
std::vector< point_int_t > | rt_candidate_cells |
std::vector< point_int_t > | rt_cells |
usec_t | sim_interval |
int | update_cb_count |
Static Public Attributes | |
static std::vector< std::string > | args |
static std::string | ctrlargs |
static const int | DEFAULT_PPM = 50 |
Protected Member Functions | |
void | CallUpdateCallbacks () |
Call all calbacks in cb_list, removing any that return true;. | |
Protected Attributes | |
std::list< std::pair < world_callback_t, void * > > | cb_list |
List of callback functions and arguments. | |
bounds3d_t | extent |
Describes the 3D volume of the world. | |
bool | graphics |
true iff we have a GUI | |
std::set< Option * > | option_table |
GUI options (toggles) registered by models. | |
std::list< PowerPack * > | powerpack_list |
List of all the powerpacks attached to models in the world. | |
usec_t | quit_time |
std::list< float * > | ray_list |
List of rays traced for debug visualization. | |
usec_t | sim_time |
the current sim time in this world in microseconds | |
std::map< point_int_t, SuperRegion * > | superregions |
std::vector< std::vector < Model * > > | update_lists |
uint64_t | updates |
the number of simulated time steps executed so far | |
Worldfile * | wf |
If set, points to the worldfile used to create this world. |
Detailed Description
World class
Constructor & Destructor Documentation
World::World | ( | const std::string & | name = "MyWorld" , |
double | ppm = DEFAULT_PPM |
||
) |
World::~World | ( | void | ) | [virtual] |
Member Function Documentation
void World::AddModel | ( | Model * | mod | ) | [virtual] |
void World::AddModelName | ( | Model * | mod, |
const std::string & | name | ||
) |
void World::AddPowerPack | ( | PowerPack * | pp | ) |
SuperRegion * World::AddSuperRegion | ( | const point_int_t & | coord | ) |
void World::AddUpdateCallback | ( | world_callback_t | cb, |
void * | user | ||
) |
Attach a callback function, to be called with the argument at the end of a complete update step
void World::CallUpdateCallbacks | ( | ) | [protected] |
Call all calbacks in cb_list, removing any that return true;.
void Stg::World::CancelQuit | ( | ) | [inline] |
Cancel a local quit request.
void Stg::World::CancelQuitAll | ( | ) | [inline] |
Cancel a global quit request.
void World::ClearRays | ( | ) |
std::string World::ClockString | ( | void | ) | const [virtual] |
Get human readable string that describes the current simulation time.
Reimplemented in Stg::WorldGui.
void World::ConsumeQueue | ( | unsigned int | queue_num | ) |
consume events from the queue up to and including the current sim_time
SuperRegion * World::CreateSuperRegion | ( | point_int_t | origin | ) |
void World::DestroySuperRegion | ( | SuperRegion * | sr | ) |
void Stg::World::DisableEnergy | ( | Model * | m | ) | [inline] |
void Stg::World::EnableEnergy | ( | Model * | m | ) | [inline] |
void Stg::World::Enqueue | ( | unsigned int | queue_num, |
usec_t | delay, | ||
Model * | mod, | ||
model_callback_t | cb, | ||
void * | arg | ||
) | [inline] |
Create a new simulation event to be handled in the future.
- Parameters:
-
queue_num Specify which queue the event should be on. The main thread is 0. delay The time from now until the event occurs, in microseconds. mod The model that should have its Update() method called at the specified time.
void World::Extend | ( | point3_t | pt | ) | [inline] |
Enlarge the bounding volume to include this point
const std::set<Model*> Stg::World::GetAllModels | ( | ) | const [inline] |
Returns a const reference to the set of models in the world.
unsigned int World::GetEventQueue | ( | Model * | mod | ) | const |
returns an event queue index number for a model to use for updates
const bounds3d_t& Stg::World::GetExtent | ( | ) | const [inline] |
Return the 3D bounding box of the world, in meters
Model* Stg::World::GetGround | ( | ) | [inline] |
Return the floor model
Model * World::GetModel | ( | const std::string & | name | ) | const |
Returns a pointer to the model identified by name, or NULL if nonexistent
SuperRegion * World::GetSuperRegion | ( | const point_int_t & | org | ) | [inline] |
SuperRegion * World::GetSuperRegionCreate | ( | const point_int_t & | org | ) | [inline] |
uint64_t Stg::World::GetUpdateCount | ( | ) | const [inline] |
Return the number of times the world has been updated.
Worldfile* Stg::World::GetWorldFile | ( | ) | [inline] |
Returns a pointer to the currently-open worlddfile object, or NULL if there is none.
virtual bool Stg::World::IsGUI | ( | ) | const [inline, virtual] |
Returns true iff this World implements a GUI. The base World class returns false, but subclasses can override this behaviour.
Reimplemented in Stg::WorldGui.
void World::Load | ( | const std::string & | worldfile_path | ) | [virtual] |
Open the file at the specified location, create a Worldfile object, read the file and configure the world from the contents, creating models as necessary. The created object persists, and can be retrieved later with World::GetWorldFile().
Reimplemented in Stg::WorldGui.
void World::LoadBlock | ( | Worldfile * | wf, |
int | entity | ||
) |
void Stg::World::LoadBlockGroup | ( | Worldfile * | wf, |
int | entity | ||
) |
void World::LoadModel | ( | Worldfile * | wf, |
int | entity | ||
) |
void World::LoadSensor | ( | Worldfile * | wf, |
int | entity | ||
) |
void World::MapPoly | ( | const std::vector< point_int_t > & | poly, |
Block * | block, | ||
unsigned int | layer | ||
) |
call Cell::AddBlock(block) for each cell on the polygon
int32_t Stg::World::MetersToPixels | ( | meters_t | x | ) | const [inline] |
convert a distance in meters to a distance in world occupancy grid pixels
point_int_t Stg::World::MetersToPixels | ( | const point_t & | pt | ) | const [inline] |
void Stg::World::NeedRedraw | ( | ) | [inline] |
hint that the world needs to be redrawn if a GUI is attached
bool World::PastQuitTime | ( | ) |
Returns true iff the current time is greater than the time we should quit
bool Stg::World::Paused | ( | ) | const [inline] |
virtual void Stg::World::PopColor | ( | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
virtual void Stg::World::PushColor | ( | Color | col | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
virtual void Stg::World::PushColor | ( | double | r, |
double | g, | ||
double | b, | ||
double | a | ||
) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
void Stg::World::Quit | ( | ) | [inline] |
Request the world quits simulation before the next timestep.
void Stg::World::QuitAll | ( | ) | [inline] |
Requests all worlds quit simulation before the next timestep.
RaytraceResult World::Raytrace | ( | const Ray & | ray | ) |
trace a ray.
RaytraceResult World::Raytrace | ( | const Pose & | pose, |
const meters_t | range, | ||
const ray_test_func_t | func, | ||
const Model * | finder, | ||
const void * | arg, | ||
const bool | ztest | ||
) |
void World::Raytrace | ( | const Pose & | pose, |
const meters_t | range, | ||
const radians_t | fov, | ||
const ray_test_func_t | func, | ||
const Model * | finder, | ||
const void * | arg, | ||
RaytraceResult * | samples, | ||
const uint32_t | sample_count, | ||
const bool | ztest | ||
) |
virtual Model* Stg::World::RecentlySelectedModel | ( | ) | const [inline, virtual] |
Reimplemented in Stg::WorldGui.
void World::RecordRay | ( | double | x1, |
double | y1, | ||
double | x2, | ||
double | y2 | ||
) |
store rays traced for debugging purposes
virtual void Stg::World::Redraw | ( | void | ) | [inline, virtual] |
Force the GUI to redraw the world, even if paused. This imlementation does nothing, but can be overridden by subclasses.
Reimplemented in Stg::WorldGui.
void World::Reload | ( | void | ) | [virtual] |
void World::RemoveModel | ( | Model * | mod | ) | [virtual] |
void World::RemovePowerPack | ( | PowerPack * | pp | ) |
int World::RemoveUpdateCallback | ( | world_callback_t | cb, |
void * | user | ||
) |
Remove a callback function. Any argument data passed to AddUpdateCallback is not automatically freed.
double Stg::World::Resolution | ( | ) | const [inline] |
Get the resolution in pixels-per-metre of the underlying discrete raytracing model
void World::Run | ( | ) | [static] |
run all worlds. If only non-gui worlds were created, UpdateAll() is repeatedly called. To simulate a gui world only a single gui world may have been created. This world is then simulated.
bool World::Save | ( | const char * | filename | ) | [virtual] |
Save the current world state into a worldfile with the given filename.
- Parameters:
-
Filename to save as.
Reimplemented in Stg::WorldGui.
void Stg::World::ShowClock | ( | bool | enable | ) | [inline] |
Control printing time to stdout.
usec_t Stg::World::SimTimeNow | ( | void | ) | const [inline] |
Returns the current simulated time in this world, in microseconds.
virtual void Stg::World::Start | ( | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
virtual void Stg::World::Stop | ( | ) | [inline, virtual] |
Reimplemented in Stg::WorldGui.
bool Stg::World::TestQuit | ( | ) | const [inline] |
virtual void Stg::World::TogglePause | ( | ) | [inline, virtual] |
void World::UnLoad | ( | ) | [virtual] |
Reimplemented in Stg::WorldGui.
bool World::Update | ( | void | ) | [virtual] |
Run one simulation timestep. Advances the simulation clock, executes all simulation updates due at the current time, then queues up future events.
Reimplemented in Stg::WorldGui.
void * World::update_thread_entry | ( | std::pair< World *, int > * | info | ) | [static] |
bool World::UpdateAll | ( | ) | [static] |
returns true when time to quit, false otherwise
uint64_t Stg::World::UpdateCount | ( | ) | [inline] |
Member Data Documentation
std::set<Model*> Stg::World::active_energy |
Set of models that require energy calculations at each World::Update().
std::set<ModelPosition*> Stg::World::active_velocity |
Set of models that require their positions to be recalculated at each World::Update().
std::vector< std::string > World::args [static] |
contains the command line arguments passed to Stg::Init(), so that controllers can read them.
std::list<std::pair<world_callback_t,void*> > Stg::World::cb_list [protected] |
List of callback functions and arguments.
std::string World::ctrlargs [static] |
const int Stg::World::DEFAULT_PPM = 50 [static] |
std::vector<std::priority_queue<Event> > Stg::World::event_queues |
Queue of pending simulation events for the main thread to handle.
bounds3d_t Stg::World::extent [protected] |
Describes the 3D volume of the world.
bool Stg::World::graphics [protected] |
true iff we have a GUI
Special model for the floor of the world
std::set<Option*> Stg::World::option_table [protected] |
GUI options (toggles) registered by models.
bool Stg::World::paused |
if true, the simulation is stopped
std::vector<std::queue<Model*> > Stg::World::pending_update_callbacks |
Queue of pending simulation events for the main thread to handle.
std::list<PowerPack*> Stg::World::powerpack_list [protected] |
List of all the powerpacks attached to models in the world.
usec_t Stg::World::quit_time [protected] |
World::quit is set true when this simulation time is reached
std::list<float*> Stg::World::ray_list [protected] |
List of rays traced for debug visualization.
std::vector<point_int_t> Stg::World::rt_candidate_cells |
std::vector<point_int_t> Stg::World::rt_cells |
The amount of simulated time to run for each call to Update()
usec_t Stg::World::sim_time [protected] |
the current sim time in this world in microseconds
std::map<point_int_t,SuperRegion*> Stg::World::superregions [protected] |
std::vector< std::vector<Model*> > Stg::World::update_lists [protected] |
uint64_t Stg::World::updates [protected] |
the number of simulated time steps executed so far
Worldfile* Stg::World::wf [protected] |
If set, points to the worldfile used to create this world.
The documentation for this class was generated from the following files:
Generated on Thu Dec 29 2011 16:43:40 for Stage by
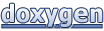