The Stage library uses its own namespace. More...
Namespaces | |
namespace | Gl |
Classes | |
class | Ancestor |
struct | blinkenlight_t |
class | Block |
class | BlockGroup |
class | Bounds |
class | bounds3d_t |
class | Camera |
class | Canvas |
class | Cell |
class | Color |
class | CProperty |
Property class. More... | |
class | CtrlArgs |
class | FileManager |
struct | fov_t |
class | Geom |
class | LogEntry |
class | Model |
Model class More... | |
class | ModelActuator |
ModelActuator class More... | |
class | ModelBlinkenlight |
class | ModelBlobfinder |
ModelBlobfinder class More... | |
class | ModelCamera |
ModelCamera class More... | |
class | ModelFiducial |
ModelFiducial class More... | |
class | ModelGripper |
class | ModelLightIndicator |
class | ModelPosition |
ModelPosition class More... | |
class | ModelRanger |
ModelRanger class More... | |
class | Option |
class | OptionsDlg |
class | OrthoCamera |
class | PerspectiveCamera |
class | point3_t |
class | point_int_t |
class | point_t |
class | Pose |
class | PowerPack |
class | Ray |
class | RaytraceResult |
class | Region |
struct | rotrect_t |
class | Size |
class | StripPlotVis |
class | SuperRegion |
class | TextureManager |
Singleton for loading textures (not threadsafe) More... | |
class | Velocity |
class | Visualizer |
class | World |
World class More... | |
class | Worldfile |
class | WorldGui |
Typedefs | |
typedef Model *(* | creator_t )(World *, Model *, const std::string &type) |
typedef uint32_t | id_t |
typedef double | joules_t |
typedef double | kg_t |
typedef double | meters_t |
typedef int(* | model_callback_t )(Model *mod, void *user) |
typedef unsigned long | msec_t |
typedef double | radians_t |
typedef bool(* | ray_test_func_t )(Model *candidate, Model *finder, const void *arg) |
typedef struct timeval | time_t |
typedef uint64_t | usec_t |
typedef double | watts_t |
typedef int(* | world_callback_t )(World *world, void *user) |
Enumerations | |
enum | { FiducialNone = 0 } |
Functions | |
const int32_t | CELLMASK (~((~0x00)<< RBITS)) |
double | constrain (double val, double minval, double maxval) |
double | dtor (double d) |
template<class T , class C > | |
void | EraseAll (T thing, C &cont) |
int32_t | GETCELL (const int32_t x) |
int32_t | GETREG (const int32_t x) |
int32_t | GETSREG (const int32_t x) |
void | Init (int *argc, char **argv[]) |
bool | InitDone () |
double | normalize (double a) |
const int32_t | RBITS (5) |
const int32_t | REGIONMASK (~((~0x00)<< SRBITS)) |
const int32_t | REGIONSIZE (REGIONWIDTH *REGIONWIDTH) |
const int32_t | REGIONWIDTH (1<< RBITS) |
void | RegisterModels () |
int | rotrects_from_image_file (const std::string &filename, std::vector< rotrect_t > &rects) |
double | rtod (double r) |
const int32_t | SBITS (5) |
int | sgn (int a) |
double | sgn (double a) |
const int32_t | SRBITS (RBITS+SBITS) |
const int32_t | SUPERREGIONSIZE (SUPERREGIONWIDTH *SUPERREGIONWIDTH) |
const int32_t | SUPERREGIONWIDTH (1<< SBITS) |
point_t * | unit_square_points_create () |
const char * | Version () |
Variables | |
const char | AUTHORS [] = "Richard Vaughan, Brian Gerkey, Andrew Howard, Reed Hedges, Pooya Karimian, Toby Collett, Jeremy Asher, Alex Couture-Beil and contributors." |
const double | billion = 1e9 |
const char | COPYRIGHT [] = "Copyright Richard Vaughan and contributors 2000-2009" |
const char | DESCRIPTION [] = "Robot simulation library\nPart of the Player Project" |
const char | LICENSE [] = "http://www.gnu.org/licenses/old-licenses/gpl-2.0.html\n" |
const double | million = 1e6 |
const double | thousand = 1e3 |
const char | WEBSITE [] = "http://playerstage.org" |
Detailed Description
The Stage library uses its own namespace.
Typedef Documentation
typedef Model*(* Stg::creator_t)(World *, Model *, const std::string &type) |
typedef uint32_t Stg::id_t |
Value that uniquely identifies a model
typedef double Stg::joules_t |
Joules: unit of energy
typedef double Stg::kg_t |
Kilograms: unit of mass
typedef double Stg::meters_t |
Metres: floating point unit of distance
typedef int(* Stg::model_callback_t)(Model *mod, void *user) |
Define a callback function type that can be attached to a record within a model and called whenever the record is set.
typedef unsigned long Stg::msec_t |
Milliseconds: unit of (short) time
typedef double Stg::radians_t |
Radians: unit of angle
typedef bool(* Stg::ray_test_func_t)(Model *candidate, Model *finder, const void *arg) |
matching function should return true iff the candidate block is stops the ray, false if the block transmits the ray
typedef struct timeval Stg::time_t |
time structure
typedef uint64_t Stg::usec_t |
Microseconds: unit of (very short) time
typedef double Stg::watts_t |
Watts: unit of power (energy/time)
typedef int(* Stg::world_callback_t)(World *world, void *user) |
Enumeration Type Documentation
Function Documentation
const int32_t Stg::CELLMASK | ( | ~ | (~0x00)<< RBITS | ) |
double Stg::constrain | ( | double | val, |
double | minval, | ||
double | maxval | ||
) |
double Stg::dtor | ( | double | d | ) | [inline] |
convert an angle in degrees to radians.
void Stg::EraseAll | ( | T | thing, |
C & | cont | ||
) |
wrapper for Erase-Remove method of removing all instances of thing from container
int32_t Stg::GETCELL | ( | const int32_t | x | ) | [inline] |
int32_t Stg::GETREG | ( | const int32_t | x | ) | [inline] |
int32_t Stg::GETSREG | ( | const int32_t | x | ) | [inline] |
void Stg::Init | ( | int * | argc, |
char ** | argv[] | ||
) |
Initialize the Stage library. Stage will parse the argument array looking for parameters in the conventnioal way.
bool Stg::InitDone | ( | ) |
returns true iff Stg::Init() has been called.
double Stg::normalize | ( | double | a | ) | [inline] |
Normalize an angle to within +/_ M_PI.
const int32_t Stg::RBITS | ( | 5 | ) |
const int32_t Stg::REGIONMASK | ( | ~ | (~0x00)<< SRBITS | ) |
const int32_t Stg::REGIONSIZE | ( | REGIONWIDTH * | REGIONWIDTH | ) |
const int32_t Stg::REGIONWIDTH | ( | 1<< | RBITS | ) |
void Stg::RegisterModels | ( | ) |
Map model names to named constructors for each model type
int Stg::rotrects_from_image_file | ( | const std::string & | filename, |
std::vector< rotrect_t > & | rects | ||
) |
load the image file [filename] and convert it to an array of rectangles, filling in the number of rects, width and height. The vector [rects] is populated with rectangles.
double Stg::rtod | ( | double | r | ) | [inline] |
convert an angle in radians to degrees.
const int32_t Stg::SBITS | ( | 5 | ) |
int Stg::sgn | ( | int | a | ) | [inline] |
take binary sign of a, either -1, or 1 if >= 0
double Stg::sgn | ( | double | a | ) | [inline] |
take binary sign of a, either -1.0, or 1.0 if >= 0.
const int32_t Stg::SRBITS | ( | RBITS+ | SBITS | ) |
const int32_t Stg::SUPERREGIONSIZE | ( | SUPERREGIONWIDTH * | SUPERREGIONWIDTH | ) |
const int32_t Stg::SUPERREGIONWIDTH | ( | 1<< | SBITS | ) |
point_t * Stg::unit_square_points_create | ( | void | ) |
create an array of 4 points containing the corners of a unit square.
const char * Stg::Version | ( | ) |
returns a human readable string indicating the libstage version number.
Variable Documentation
const char Stg::AUTHORS[] = "Richard Vaughan, Brian Gerkey, Andrew Howard, Reed Hedges, Pooya Karimian, Toby Collett, Jeremy Asher, Alex Couture-Beil and contributors." |
Author string
const double Stg::billion = 1e9 |
Convenient constant
const char Stg::COPYRIGHT[] = "Copyright Richard Vaughan and contributors 2000-2009" |
Copyright string
const char Stg::DESCRIPTION[] = "Robot simulation library\nPart of the Player Project" |
Project description string
const char Stg::LICENSE[] = "http://www.gnu.org/licenses/old-licenses/gpl-2.0.html\n" |
Project distribution license string
const double Stg::million = 1e6 |
Convenient constant
const double Stg::thousand = 1e3 |
Convenient constant
const char Stg::WEBSITE[] = "http://playerstage.org" |
Project website string
Generated on Thu Dec 29 2011 16:43:39 for Stage by
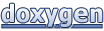