Model class More...
#include <stage.hh>
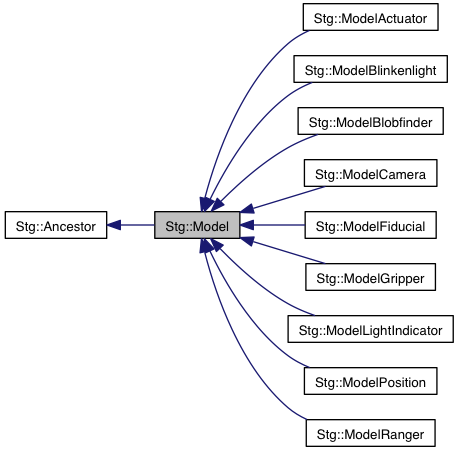
Classes | |
class | cb_t |
class | Flag |
class | GuiState |
class | RasterVis |
class | TrailItem |
class | Visibility |
Public Types | |
enum | callback_type_t { CB_FLAGDECR, CB_FLAGINCR, CB_GEOM, CB_INIT, CB_LOAD, CB_PARENT, CB_POSE, CB_SAVE, CB_SHUTDOWN, CB_STARTUP, CB_UPDATE, CB_VELOCITY, __CB_TYPE_COUNT } |
Public Member Functions | |
Block * | AddBlockRect (meters_t x, meters_t y, meters_t dx, meters_t dy, meters_t dz) |
void | AddCallback (callback_type_t type, model_callback_t cb, void *user) |
void | AddFlag (Flag *flag) |
void | AddToPose (const Pose &pose) |
void | AddToPose (double dx, double dy, double dz, double da) |
void | AddVisualizer (Visualizer *custom_visual, bool on_by_default) |
void | BecomeParentOf (Model *child) |
int | CallCallbacks (callback_type_t type) |
void | ClearBlocks () |
bool | DataIsFresh () const |
void | Disable () |
void | Enable () |
PowerPack * | FindPowerPack () const |
Model * | GetChild (const std::string &name) const |
Color | GetColor () const |
usec_t | GetEnergyInterval () const |
int | GetFiducialReturn () const |
unsigned int | GetFlagCount () const |
Geom | GetGeom () const |
Pose | GetGlobalPose () const |
Velocity | GetGlobalVelocity () const |
uint32_t | GetId () const |
usec_t | GetInterval () |
kg_t | GetMassOfChildren () const |
const std::string & | GetModelType () const |
Pose | GetPose () const |
std::string | GetSayString () |
unsigned int | GetSubscriptionCount () const |
kg_t | GetTotalMass () const |
Model * | GetUnsubscribedModelOfType (const std::string &type) const |
Model * | GetUnusedModelOfType (const std::string &type) |
usec_t | GetUpdateInterval () const |
World * | GetWorld () const |
Pose | GlobalToLocal (const Pose &pose) const |
bool | HasSubscribers () const |
void | InitControllers () |
bool | IsAntecedent (const Model *testmod) const |
bool | IsDescendent (const Model *testmod) const |
bool | IsRelated (const Model *testmod) const |
void | Load (Worldfile *wf, int wf_entity) |
virtual void | Load () |
void | LoadBlock (Worldfile *wf, int entity) |
void | LoadControllerModule (const char *lib) |
Pose | LocalToGlobal (const Pose &pose) const |
point_t | LocalToGlobal (const point_t &pt) const |
std::vector< point_int_t > | LocalToPixels (const std::vector< point_t > &local) const |
Model (World *world, Model *parent=NULL, const std::string &type="model", const std::string &name="") | |
Model () | |
void | NeedRedraw () |
Model * | Parent () const |
void | PlaceInFreeSpace (meters_t xmin, meters_t xmax, meters_t ymin, meters_t ymax) |
virtual void | PopColor () |
Flag * | PopFlag () |
std::string | PoseString () |
virtual void | Print (char *prefix) const |
virtual const char * | PrintWithPose () const |
virtual void | PushColor (Color col) |
virtual void | PushColor (double r, double g, double b, double a) |
void | PushFlag (Flag *flag) |
void | Rasterize (uint8_t *data, unsigned int width, unsigned int height, meters_t cellwidth, meters_t cellheight) |
void | Redraw () |
int | RemoveCallback (callback_type_t type, model_callback_t callback) |
void | RemoveFlag (Flag *flag) |
void | RemoveVisualizer (Visualizer *custom_visual) |
Model * | Root () |
virtual void | Save () |
void | Say (const std::string &str) |
void | SetBlobReturn (bool val) |
void | SetBoundary (bool val) |
void | SetColor (Color col) |
void | SetFiducialKey (int key) |
void | SetFiducialReturn (int fid) |
void | SetFriction (double friction) |
void | SetGeom (const Geom &src) |
void | SetGlobalPose (const Pose &gpose) |
void | SetGlobalVelocity (const Velocity &gvel) |
void | SetGravityReturn (bool val) |
void | SetGripperReturn (bool val) |
void | SetGuiGrid (bool val) |
void | SetGuiMove (bool val) |
void | SetGuiNose (bool val) |
void | SetGuiOutline (bool val) |
void | SetMapResolution (meters_t res) |
void | SetMass (kg_t mass) |
void | SetObstacleReturn (bool val) |
int | SetParent (Model *newparent) |
void | SetPose (const Pose &pose) |
void | SetRangerReturn (double val) |
void | SetRangerReturn (bool val) |
void | SetStall (bool stall) |
void | SetStickyReturn (bool val) |
virtual void | SetToken (const std::string &str) |
void | SetWatts (watts_t watts) |
void | SetWorldfile (Worldfile *wf, int wf_entity) |
bool | Stalled () const |
void | Subscribe () |
void | Unsubscribe () |
virtual | ~Model () |
Static Public Member Functions | |
static Model * | LookupId (uint32_t id) |
Public Attributes | |
class Stg::Model::Visibility | vis |
Static Public Attributes | |
static std::map< std::string, creator_t > | name_map |
Protected Member Functions | |
void | AppendTouchingModels (std::set< Model * > &touchers) |
void | CallUpdateCallbacks (void) |
void | CommitTestedPose () |
virtual void | DataVisualize (Camera *cam) |
void | DataVisualizeTree (Camera *cam) |
virtual void | DrawBlocks () |
void | DrawBlocksTree () |
void | DrawBoundingBox () |
void | DrawBoundingBoxTree () |
void | DrawFlagList () |
void | DrawGrid () |
void | DrawImage (uint32_t texture_id, Camera *cam, float alpha, double width=1.0, double height=1.0) |
void | DrawOrigin () |
void | DrawOriginTree () |
virtual void | DrawPicker () |
void | DrawPose (Pose pose) |
virtual void | DrawSelected (void) |
virtual void | DrawStatus (Camera *cam) |
void | DrawStatusTree (Camera *cam) |
void | DrawTrailArrows () |
void | DrawTrailBlocks () |
void | DrawTrailFootprint () |
void | Map (unsigned int layer) |
void | Map () |
void | MapFromRoot (unsigned int layer) |
void | MapWithChildren (unsigned int layer) |
meters_t | ModelHeight () const |
void | PopCoords () |
void | PushLocalCoords () |
RaytraceResult | Raytrace (const Pose &pose, const meters_t range, const ray_test_func_t func, const void *arg, const bool ztest=true) |
void | Raytrace (const Pose &pose, const meters_t range, const radians_t fov, const ray_test_func_t func, const void *arg, RaytraceResult *samples, const uint32_t sample_count, const bool ztest=true) |
RaytraceResult | Raytrace (const radians_t bearing, const meters_t range, const ray_test_func_t func, const void *arg, const bool ztest=true) |
void | Raytrace (const radians_t bearing, const meters_t range, const radians_t fov, const ray_test_func_t func, const void *arg, RaytraceResult *samples, const uint32_t sample_count, const bool ztest=true) |
void | RegisterOption (Option *opt) |
virtual void | Shutdown () |
virtual void | Startup () |
Model * | TestCollision () |
void | UnMap (unsigned int layer) |
void | UnMap () |
void | UnMapFromRoot (unsigned int layer) |
void | UnMapWithChildren (unsigned int layer) |
virtual void | Update () |
virtual void | UpdateCharge () |
void | UpdateTrail () |
Static Protected Member Functions | |
static int | UpdateWrapper (Model *mod, void *arg) |
Protected Attributes | |
bool | alwayson |
BlockGroup | blockgroup |
int | blocks_dl |
int | boundary |
std::vector< std::set< cb_t > > | callbacks |
Color | color |
std::list< Visualizer * > | cv_list |
bool | data_fresh |
bool | disabled |
unsigned int | event_queue_num |
std::list< Flag * > | flag_list |
double | friction |
Geom | geom |
class Stg::Model::GuiState | gui |
bool | has_default_block |
uint32_t | id |
usec_t | interval |
time between updates in usec | |
usec_t | interval_energy |
time between updates of powerpack in usec | |
usec_t | last_update |
time of last update in us | |
bool | log_state |
iff true, model state is logged | |
meters_t | map_resolution |
kg_t | mass |
Model * | parent |
Pose | pose |
PowerPack * | power_pack |
std::list< PowerPack * > | pps_charging |
Stg::Model::RasterVis | rastervis |
bool | rebuild_displaylist |
iff true, regenerate block display list before redraw | |
std::string | say_string |
if non-empty, this string is displayed in the GUI | |
bool | stack_children |
whether child models should be stacked on top of this model or not | |
bool | stall |
Set to true iff the model collided with something else. | |
int | subs |
the number of subscriptions to this model | |
bool | thread_safe |
std::vector< TrailItem > | trail |
unsigned int | trail_index |
const std::string | type |
bool | used |
TRUE iff this model has been returned by GetUnusedModelOfType() | |
watts_t | watts |
power consumed by this model | |
watts_t | watts_give |
watts_t | watts_take |
Worldfile * | wf |
int | wf_entity |
World * | world |
WorldGui * | world_gui |
Static Protected Attributes | |
static uint64_t | trail_interval |
static unsigned int | trail_length |
Detailed Description
Model class
Member Enumeration Documentation
Constructor & Destructor Documentation
Model::Model | ( | World * | world, |
Model * | parent = NULL , |
||
const std::string & | type = "model" , |
||
const std::string & | name = "" |
||
) |
Constructor
Model::~Model | ( | void | ) | [virtual] |
Destructor
Stg::Model::Model | ( | ) | [inline] |
Alternate constructor that creates dummy models with only a pose
Member Function Documentation
Add a block to this model centered at [x,y] with extent [dx, dy, dz]
void Model::AddCallback | ( | callback_type_t | type, |
model_callback_t | cb, | ||
void * | user | ||
) |
Add a callback. The specified function is called whenever the indicated model method is called, and passed the user data.
- Parameters:
-
cb Pointer the function to be called. user Pointer to arbitrary user data, passed to the callback when called.
void Model::AddFlag | ( | Flag * | flag | ) |
void Model::AddToPose | ( | const Pose & | pose | ) |
add values to a model's pose in its parent's coordinate system
void Model::AddToPose | ( | double | dx, |
double | dy, | ||
double | dz, | ||
double | da | ||
) |
add values to a model's pose in its parent's coordinate system
void Model::AddVisualizer | ( | Visualizer * | custom_visual, |
bool | on_by_default | ||
) |
Attach a user supplied visualization to a model.
void Model::AppendTouchingModels | ( | std::set< Model * > & | touchers | ) | [protected] |
void Model::BecomeParentOf | ( | Model * | child | ) |
int Model::CallCallbacks | ( | callback_type_t | type | ) |
void Model::CallUpdateCallbacks | ( | void | ) | [protected] |
Calls CallCallback( CB_UPDATE )
void Model::ClearBlocks | ( | ) |
remove all blocks from this model, freeing their memory
void Stg::Model::CommitTestedPose | ( | ) | [protected] |
bool Stg::Model::DataIsFresh | ( | ) | const [inline] |
void Model::DataVisualize | ( | Camera * | cam | ) | [protected, virtual] |
Reimplemented in Stg::ModelCamera, and Stg::ModelBlinkenlight.
void Model::DataVisualizeTree | ( | Camera * | cam | ) | [protected] |
void Stg::Model::Disable | ( | ) | [inline] |
Disable the model. Its pose will not change due to velocity until re-enabled using Enable(). This is used for example when dragging a model with the mouse pointer. The model is enabled by default.
void Model::DrawBlocks | ( | ) | [protected, virtual] |
Reimplemented in Stg::ModelLightIndicator.
void Model::DrawBlocksTree | ( | ) | [protected] |
void Model::DrawBoundingBox | ( | ) | [protected] |
void Model::DrawBoundingBoxTree | ( | ) | [protected] |
void Model::DrawFlagList | ( | void | ) | [protected] |
void Model::DrawGrid | ( | void | ) | [protected] |
void Model::DrawImage | ( | uint32_t | texture_id, |
Camera * | cam, | ||
float | alpha, | ||
double | width = 1.0 , |
||
double | height = 1.0 |
||
) | [protected] |
Draw the image stored in texture_id above the model
void Stg::Model::DrawOrigin | ( | ) | [protected] |
void Model::DrawOriginTree | ( | ) | [protected] |
void Model::DrawPicker | ( | void | ) | [protected, virtual] |
void Model::DrawPose | ( | Pose | pose | ) | [protected] |
void Model::DrawSelected | ( | void | ) | [protected, virtual] |
void Model::DrawStatus | ( | Camera * | cam | ) | [protected, virtual] |
void Model::DrawStatusTree | ( | Camera * | cam | ) | [protected] |
void Model::DrawTrailArrows | ( | ) | [protected] |
void Model::DrawTrailBlocks | ( | ) | [protected] |
void Model::DrawTrailFootprint | ( | ) | [protected] |
void Stg::Model::Enable | ( | ) | [inline] |
Enable the model, so that non-zero velocities will change the model's pose. Models are enabled by default.
PowerPack * Model::FindPowerPack | ( | ) | const |
Model * Model::GetChild | ( | const std::string & | name | ) | const |
Returns a pointer to the model identified by name, or NULL if it doesn't exist in this model.
Color Stg::Model::GetColor | ( | ) | const [inline] |
usec_t Stg::Model::GetEnergyInterval | ( | ) | const [inline] |
int Stg::Model::GetFiducialReturn | ( | ) | const [inline] |
Get a model's fiducial return value.
unsigned int Stg::Model::GetFlagCount | ( | ) | const [inline] |
Geom Stg::Model::GetGeom | ( | ) | const [inline] |
Get (a copy of) the model's geometry - it's size and local pose (offset from origin in local coords).
Pose Model::GetGlobalPose | ( | ) | const [virtual] |
get the pose of a model in the global CS
Reimplemented from Stg::Ancestor.
Velocity Stg::Model::GetGlobalVelocity | ( | ) | const |
get the velocity of a model in the global CS
uint32_t Stg::Model::GetId | ( | ) | const [inline] |
return a model's unique process-wide identifier
usec_t Stg::Model::GetInterval | ( | ) | [inline] |
return the update interval in usec
kg_t Model::GetMassOfChildren | ( | ) | const |
Get the mass of this model's children recursively.
const std::string& Stg::Model::GetModelType | ( | ) | const [inline] |
Pose Stg::Model::GetPose | ( | ) | const [inline] |
Get (a copy of) the pose of a model in its parent's coordinate system.
std::string Stg::Model::GetSayString | ( | ) | [inline] |
unsigned int Stg::Model::GetSubscriptionCount | ( | ) | const [inline] |
Returns the current number of subscriptions. If alwayson, this is never less than 1.
kg_t Model::GetTotalMass | ( | ) | const |
Get the total mass of a model and it's children recursively
Model * Model::GetUnsubscribedModelOfType | ( | const std::string & | type | ) | const |
returns the first descendent of this model that is unsubscribed and has the type indicated by the string
Model * Model::GetUnusedModelOfType | ( | const std::string & | type | ) |
returns the first descendent of this model that is unused and has the type indicated by the string. This model is tagged as used.
usec_t Stg::Model::GetUpdateInterval | ( | ) | const [inline] |
World* Stg::Model::GetWorld | ( | ) | const [inline] |
Returns a pointer to the world that contains this model
Given a global pose, returns that pose in the model's local coordinate system.
bool Stg::Model::HasSubscribers | ( | ) | const [inline] |
Returns true if the model has one or more subscribers, else false.
void Model::InitControllers | ( | ) |
Call Init() for all attached controllers.
bool Model::IsAntecedent | ( | const Model * | testmod | ) | const |
bool Model::IsDescendent | ( | const Model * | testmod | ) | const |
returns true if model [testmod] is a descendent of this model
bool Model::IsRelated | ( | const Model * | testmod | ) | const |
returns true if model [testmod] is a descendent or antecedent of this model
void Stg::Model::Load | ( | Worldfile * | wf, |
int | wf_entity | ||
) | [inline] |
Set the worldfile and worldfile entity ID - must be called before Load()
Reimplemented from Stg::Ancestor.
void Model::Load | ( | void | ) | [virtual] |
configure a model by reading from the current world file
Reimplemented in Stg::ModelActuator, Stg::ModelPosition, Stg::ModelCamera, Stg::ModelBlinkenlight, Stg::ModelRanger, Stg::ModelFiducial, Stg::ModelGripper, and Stg::ModelBlobfinder.
void Model::LoadBlock | ( | Worldfile * | wf, |
int | entity | ||
) |
Add a block to this model by loading it from a worldfile entity
void Model::LoadControllerModule | ( | const char * | lib | ) |
Load a control program for this model from an external library
Return the global pose (i.e. pose in world coordinates) of a pose specified in the model's local coordinate system
Return the 2d point in world coordinates of a 2d point specified in the model's local coordinate system
std::vector< point_int_t > Model::LocalToPixels | ( | const std::vector< point_t > & | local | ) | const |
Return a vector of global pixels corresponding to a vector of local points.
static Model* Stg::Model::LookupId | ( | uint32_t | id | ) | [inline, static] |
Look up a model pointer by a unique model ID
void Model::Map | ( | unsigned int | layer | ) | [protected] |
void Stg::Model::Map | ( | ) | [inline, protected] |
Call Map on all layers
void Model::MapFromRoot | ( | unsigned int | layer | ) | [protected] |
void Model::MapWithChildren | ( | unsigned int | layer | ) | [protected] |
meters_t Model::ModelHeight | ( | ) | const [protected] |
void Model::NeedRedraw | ( | void | ) |
Sets the redraw flag, so this model will be redrawn at the earliest opportunity
Model* Stg::Model::Parent | ( | ) | const [inline] |
Returns a pointer to this model's parent model, or NULL if this model has no parent
virtual void Stg::Model::PopColor | ( | ) | [inline, virtual] |
void Model::PopCoords | ( | ) | [protected] |
Model::Flag * Model::PopFlag | ( | ) |
std::string Stg::Model::PoseString | ( | ) | [inline] |
Return a human-readable string describing the model's pose
void Model::Print | ( | char * | prefix | ) | const [virtual] |
Reimplemented in Stg::ModelRanger.
const char * Model::PrintWithPose | ( | ) | const [virtual] |
virtual void Stg::Model::PushColor | ( | Color | col | ) | [inline, virtual] |
virtual void Stg::Model::PushColor | ( | double | r, |
double | g, | ||
double | b, | ||
double | a | ||
) | [inline, virtual] |
void Model::PushFlag | ( | Flag * | flag | ) |
void Model::PushLocalCoords | ( | ) | [protected] |
void Model::Rasterize | ( | uint8_t * | data, |
unsigned int | width, | ||
unsigned int | height, | ||
meters_t | cellwidth, | ||
meters_t | cellheight | ||
) |
Render the model's blocks as an occupancy grid into the preallocated array of width by height pixels
RaytraceResult Model::Raytrace | ( | const Pose & | pose, |
const meters_t | range, | ||
const ray_test_func_t | func, | ||
const void * | arg, | ||
const bool | ztest = true |
||
) | [protected] |
raytraces a single ray from the point and heading identified by pose, in local coords
void Stg::Model::Raytrace | ( | const Pose & | pose, |
const meters_t | range, | ||
const radians_t | fov, | ||
const ray_test_func_t | func, | ||
const void * | arg, | ||
RaytraceResult * | samples, | ||
const uint32_t | sample_count, | ||
const bool | ztest = true |
||
) | [protected] |
raytraces multiple rays around the point and heading identified by pose, in local coords
RaytraceResult Model::Raytrace | ( | const radians_t | bearing, |
const meters_t | range, | ||
const ray_test_func_t | func, | ||
const void * | arg, | ||
const bool | ztest = true |
||
) | [protected] |
void Model::Raytrace | ( | const radians_t | bearing, |
const meters_t | range, | ||
const radians_t | fov, | ||
const ray_test_func_t | func, | ||
const void * | arg, | ||
RaytraceResult * | samples, | ||
const uint32_t | sample_count, | ||
const bool | ztest = true |
||
) | [protected] |
void Model::Redraw | ( | void | ) |
Force the GUI (if any) to redraw this model
int Model::RemoveCallback | ( | callback_type_t | type, |
model_callback_t | callback | ||
) |
void Model::RemoveFlag | ( | Flag * | flag | ) |
void Model::RemoveVisualizer | ( | Visualizer * | custom_visual | ) |
remove user supplied visualization to a model - supply the same ptr passed to AddCustomVisualizer
Model* Stg::Model::Root | ( | ) | [inline] |
return the root model of the tree containing this model
void Model::Save | ( | void | ) | [virtual] |
save the state of the model to the current world file
Reimplemented in Stg::ModelGripper.
void Model::Say | ( | const std::string & | str | ) |
void Model::SetBlobReturn | ( | bool | val | ) |
void Model::SetBoundary | ( | bool | val | ) |
void Model::SetColor | ( | Color | col | ) |
void Model::SetFiducialKey | ( | int | key | ) |
set a model's fiducial key: only fiducial finders with a matching key can detect this model as a fiducial.
void Model::SetFiducialReturn | ( | int | fid | ) |
Set a model's fiducial return value. Values less than zero are not detected by the fiducial sensor.
void Model::SetFriction | ( | double | friction | ) |
void Model::SetGeom | ( | const Geom & | src | ) |
set a model's geometry (size and center offsets)
void Model::SetGlobalPose | ( | const Pose & | gpose | ) |
set the pose of model in global coordinates
void Stg::Model::SetGlobalVelocity | ( | const Velocity & | gvel | ) |
void Stg::Model::SetGravityReturn | ( | bool | val | ) |
void Model::SetGripperReturn | ( | bool | val | ) |
void Model::SetGuiGrid | ( | bool | val | ) |
void Model::SetGuiMove | ( | bool | val | ) |
void Model::SetGuiNose | ( | bool | val | ) |
void Model::SetGuiOutline | ( | bool | val | ) |
void Model::SetMapResolution | ( | meters_t | res | ) |
void Model::SetMass | ( | kg_t | mass | ) |
void Model::SetObstacleReturn | ( | bool | val | ) |
int Model::SetParent | ( | Model * | newparent | ) |
Change a model's parent - experimental
void Model::SetPose | ( | const Pose & | pose | ) |
Enable update of model pose according to velocity state Disable update of model pose according to velocity state set a model's pose in its parent's coordinate system
void Model::SetRangerReturn | ( | double | val | ) |
void Stg::Model::SetRangerReturn | ( | bool | val | ) |
void Model::SetStall | ( | bool | stall | ) |
void Stg::Model::SetStickyReturn | ( | bool | val | ) |
virtual void Stg::Model::SetToken | ( | const std::string & | str | ) | [inline, virtual] |
Reimplemented from Stg::Ancestor.
void Model::SetWatts | ( | watts_t | watts | ) |
void Stg::Model::SetWorldfile | ( | Worldfile * | wf, |
int | wf_entity | ||
) | [inline] |
Set the worldfile and worldfile entity ID
void Model::Shutdown | ( | void | ) | [protected, virtual] |
Reimplemented in Stg::ModelActuator, Stg::ModelPosition, Stg::ModelRanger, Stg::ModelFiducial, and Stg::ModelBlobfinder.
bool Stg::Model::Stalled | ( | ) | const [inline] |
Returns the value of the model's stall boolean, which is true iff the model has crashed into another model
void Model::Startup | ( | void | ) | [protected, virtual] |
Reimplemented in Stg::ModelActuator, Stg::ModelPosition, Stg::ModelRanger, and Stg::ModelBlobfinder.
void Model::Subscribe | ( | void | ) |
subscribe to a model's data
Model * Model::TestCollision | ( | ) | [protected] |
Check to see if the current pose will yield a collision with obstacles. Returns a pointer to the first entity we are in collision with, or NULL if no collision exists. Recursively calls TestCollision() on all descendents.
void Model::UnMap | ( | unsigned int | layer | ) | [protected] |
void Stg::Model::UnMap | ( | ) | [inline, protected] |
Call UnMap on all layers
void Model::UnMapFromRoot | ( | unsigned int | layer | ) | [protected] |
void Model::UnMapWithChildren | ( | unsigned int | layer | ) | [protected] |
void Model::Unsubscribe | ( | void | ) |
unsubscribe from a model's data
void Model::Update | ( | void | ) | [protected, virtual] |
Reimplemented in Stg::ModelActuator, Stg::ModelPosition, Stg::ModelCamera, Stg::ModelBlinkenlight, Stg::ModelRanger, and Stg::ModelBlobfinder.
void Model::UpdateCharge | ( | ) | [protected, virtual] |
void Model::UpdateTrail | ( | ) | [protected] |
Record the current pose in our trail. Delete the trail head if it is full.
static int Stg::Model::UpdateWrapper | ( | Model * | mod, |
void * | arg | ||
) | [inline, static, protected] |
Member Data Documentation
bool Stg::Model::alwayson [protected] |
If true, the model always has at least one subscription, so always runs. Defaults to false.
BlockGroup Stg::Model::blockgroup [protected] |
int Stg::Model::blocks_dl [protected] |
OpenGL display list identifier for the blockgroup
int Stg::Model::boundary [protected] |
Iff true, 4 thin blocks are automatically added to the model, forming a solid boundary around the bounding box of the model.
std::vector<std::set<cb_t> > Stg::Model::callbacks [protected] |
A list of callback functions can be attached to any address. When Model::CallCallbacks( void*) is called, the callbacks are called.
Color Stg::Model::color [protected] |
Default color of the model's blocks.
std::list<Visualizer*> Stg::Model::cv_list [protected] |
Container for Visualizers attached to this model.
bool Stg::Model::data_fresh [protected] |
This can be set to indicate that the model has new data that may be of interest to users. This allows polling the model instead of adding a data callback.
bool Stg::Model::disabled [protected] |
If set true, Update() is not called on this model. Useful e.g. for temporarily disabling updates when dragging models with the mouse.
unsigned int Stg::Model::event_queue_num [protected] |
The index into the world's vector of event queues. Initially -1, to indicate that it is not on a list yet.
std::list<Flag*> Stg::Model::flag_list [protected] |
Container for flags attached to this model.
double Stg::Model::friction [protected] |
Geom Stg::Model::geom [protected] |
Specifies the the size of this model's bounding box, and the offset of its local coordinate system wrt that its parent.
class Stg::Model::GuiState Stg::Model::gui [protected] |
bool Stg::Model::has_default_block [protected] |
uint32_t Stg::Model::id [protected] |
unique process-wide identifier for this model
usec_t Stg::Model::interval [protected] |
time between updates in usec
usec_t Stg::Model::interval_energy [protected] |
time between updates of powerpack in usec
usec_t Stg::Model::last_update [protected] |
time of last update in us
bool Stg::Model::log_state [protected] |
iff true, model state is logged
meters_t Stg::Model::map_resolution [protected] |
kg_t Stg::Model::mass [protected] |
std::map< std::string, creator_t > Model::name_map [static] |
Model* Stg::Model::parent [protected] |
Pointer to the parent of this model, possibly NULL.
Pose Stg::Model::pose [protected] |
The pose of the model in it's parents coordinate frame, or the global coordinate frame is the parent is NULL.
PowerPack* Stg::Model::power_pack [protected] |
Optional attached PowerPack, defaults to NULL
std::list<PowerPack*> Stg::Model::pps_charging [protected] |
list of powerpacks that this model is currently charging, initially NULL.
Stg::Model::RasterVis Stg::Model::rastervis [protected] |
bool Stg::Model::rebuild_displaylist [protected] |
iff true, regenerate block display list before redraw
std::string Stg::Model::say_string [protected] |
if non-empty, this string is displayed in the GUI
bool Stg::Model::stack_children [protected] |
whether child models should be stacked on top of this model or not
bool Stg::Model::stall [protected] |
Set to true iff the model collided with something else.
int Stg::Model::subs [protected] |
the number of subscriptions to this model
bool Stg::Model::thread_safe [protected] |
Thread safety flag. Iff true, Update() may be called in parallel with other models. Defaults to false for safety. Derived classes can set it true in their constructor to allow parallel Updates().
std::vector<TrailItem> Stg::Model::trail [protected] |
a ring buffer for storing recent poses
unsigned int Stg::Model::trail_index [protected] |
current position in the ring buffer
uint64_t Model::trail_interval [static, protected] |
Number of world updates between trail records.
uint32_t Model::trail_length [static, protected] |
The maxiumum length of the trail drawn. Default is 20, but can be set in the world file using the tail_length model property.
const std::string Stg::Model::type [protected] |
bool Stg::Model::used [protected] |
TRUE iff this model has been returned by GetUnusedModelOfType()
Reimplemented in Stg::ModelRanger, and Stg::ModelBlobfinder.
watts_t Stg::Model::watts [protected] |
power consumed by this model
watts_t Stg::Model::watts_give [protected] |
If >0, this model can transfer energy to models that have watts_take >0
watts_t Stg::Model::watts_take [protected] |
If >0, this model can transfer energy from models that have watts_give >0
Worldfile* Stg::Model::wf [protected] |
int Stg::Model::wf_entity [protected] |
World* Stg::Model::world [protected] |
WorldGui* Stg::Model::world_gui [protected] |
The documentation for this class was generated from the following files:
Generated on Thu Dec 29 2011 16:43:40 for Stage by
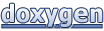